Using .NET MAUI Community Toolkit
The .NET Community Toolkit recently debuted. Let's take a look at how to use some of it's features, and how we can contribute more!
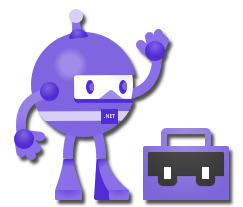
Installing the .NET MAUI Community Toolkits
There are currently two Community Toolkits focused on .NET MAUI:
- CommunityToolkit.Maui
- A collection of Animations, Behaviors, Converters and UI Controls for development with .NET MAUI. It simplifies and demonstrates common developer tools building iOS, Android, macOS and Windows apps with .NET MAUI
- Sample App: https://github.com/brminnick/HelloMauiToolkit
- CommunityToolkit.Maui.Markup
- A set of fluent helper methods to simplify building declarative .NET MAUI user interfaces in C#
- Sample App: https://github.com/brminnick/HelloMauiMarkup
Both NuGet Packages can be added to any project targeting .NET 6 or later:
- Open a
net6.0
project in Visual Studio - In the Visual Studio Package Manager Console, enter the following command:
Install-Package CommunityToolkit.Maui
or
Install-Package CommunityToolkit.Maui.Markup
Converters
The Converters found in ComunityToolkit.Maui
can be easiliy added to our MVVM Bindings allowing us to easily convert the value between our ViewModel and our View.
Some of the popular converters include:
InvertedBoolConverter
ListToStringConverter
TextCaseConverter
- ...and more!
Check out this sample app to see how to use CommunityToolkit.Maui.Converters
: https://github.com/brminnick/HelloMauiToolkit
Using Converters in .NET MAUI Community Toolkit, XAML
-
Install the .NET MAUI Community Toolkit NuGet Package
Install-Package CommunityToolkit.Maui
-
In XAML, add the following XML Namespace:
xmlns:converters="clr-namespace:CommunityToolkit.Maui.Converters;assembly=CommunityToolkit.Maui"
-
In XAML, add the converter to your
Binding
<Button Text="Get Data" IsEnabled="{Binding Path=IsBusy, Converter={converters:InvertedBoolConverter}} />
Using Converters in .NET MAUI Community Toolkit, C#
Note: The following example uses the C# Markup Extensions found in .NET MAUI Markup Community Toolkit
-
Install the .NET MAUI Community Toolkit + .NET MAUI Markup Community Toolkit NuGet Packages
Install-Package CommunityToolkit.Maui Install-Package CommunityToolkit.Maui.Markup
-
In C#, add the following namespaces:
using CommunityToolkit.Maui; using CommunityToolkit.Maui.Markup;
-
In C#, add the converter to your
Binding
new Button().Bind(Button.IsVisibleProperty, nameof(ViewModel.IsBusy), converter: new InvertedBoolConverter())
Behaviors
The Behaviors are found in ComunityToolkit.Maui
and let us add functionality to user interface controls without having to subclass them.
Some of the popular converters include:
EventToCommandBehavior
UserStoppedTypingBehavior
- ...and more!
Using Behaviors with .NET MAUI Community Toolkit, XAML
-
Install the .NET MAUI Community Toolkit NuGet Package
Install-Package CommunityToolkit.Maui
-
In XAML, add the following XML Namespace:
xmlns:behaviors="clr-namespace:CommunityToolkit.Maui.Behaviors;assembly=CommunityToolkit.Maui"
-
In XAML, add the Behavior to your UI
<SearchBar Placeholder="Search..." > <SearchBar.Behaviors> <behaviors:UserStoppedTypingBehavior Command="{Binding SearchCommand}" StoppedTypingTimeThreshold="1000" MinimumLengthThreshold="3" ShouldDismissKeyboardAutomatically="True" /> </SearchBar.Behaviors> </SearchBar>
Using Behaviors with .NET MAUI Community Toolkit, C#
Note: The following example uses the C# Markup Extensions found in .NET MAUI Markup Community Toolkit
-
Install the .NET MAUI Community Toolkit + .NET MAUI Markup Community Toolkit NuGet Packages
Install-Package CommunityToolkit.Maui Install-Package CommunityToolkit.Maui.Markup
-
In C#, add the following namespaces:
using CommunityToolkit.Maui; using CommunityToolkit.Maui.Markup;
-
In C#, add the converter to your
Binding
var userStoppedTypingBehavior = new UserStoppedTypingBehavior { MinimumLengthThreshold = 3, StoppedTypingTimeThreshold = 1000, ShouldDismissKeyboardAutomatically = true }.Bind(UserStoppedTypingBehavior.CommandProperty, nameof(ViewModel.SearchCommand)); var searchBar = new SearchBar(); searchBar.Behaviors.Add(userStoppedTypingBehavior);
C# Markup Extensions
For .NET MAUI developers who prefer to write UI in C# instead of XAML, the .NET MAUI Markup Community Toolkit makes life A LOT easier!
The .NET MAUI Markup Community Toolkit is a collection of Fluent C# Extension Methods that allows developers to continue architecting their apps using MVVM, Bindings, Resource Dictionaries, etc., without the need for XAML
Check out this sample app to see how to use CommunityToolkit.Maui.Markup
: https://github.com/brminnick/HelloMauiMarkup
Creating a Grid with .NET MAUI Markup Community Toolkit
With C# Markup Markup extensions, we can define our Rows + Columns with a custom Enum
and then use the fluent C# extensions methods to create our Grid
!
using System;
using CommunityToolkit.Maui.Markup;
using Microsoft.Maui;
using Microsoft.Maui.Controls;
using Microsoft.Maui.Essentials;
using static CommunityToolkit.Maui.Markup.GridRowsColumns;
namespace HelloMauiMarkup;
class MainPage : ContentPge
{
public MainPage()
{
BindingContext = new MainViewModel();
Content = new Grid
{
RowSpacing = 25,
ColumnSpacing = 0,
Padding = Device.RuntimePlatform switch
{
Device.iOS => new Thickness(30, 60, 30, 30),
_ => new Thickness(30)
},
RowDefinitions = Rows.Define(
(Row.HelloWorld, 44),
(Row.Welcome, Auto),
(Row.Count, Auto),
(Row.ClickMeButton, Auto),
(Row.Image, Star)),
ColumnDefinitions = Columns.Define(
(Column.Text, Star),
(Column.Number, Star)),
Children =
{
new Label { Text = "Hello World" }
.Row(Row.HelloWorld).ColumnSpan(All<Column>())
.Font(size: 32)
.CenterHorizontal().TextCenter(),
new Label { Text = "Welcome to .NET MAUI Markup Community Toolkit Sample" }
.Row(Row.Welcome).ColumnSpan(All<Column>())
.Font(size: 18)
.CenterHorizontal().TextCenter(),
new Label { Text = "Current Count: " }
.Row(Row.Count).Column(Column.Text)
.Font(bold: true)
.End().TextEnd(),
new Label()
.Row(Row.Count).Column(Column.Number)
.Font(bold: true)
.Start().TextStart()
.Bind<Label, int, string>(Label.TextProperty, nameof(MainViewModel.ClickCount), convert: count => count.ToString())
new Button { Text = "Click Me" }
.Row(Row.ClickMeButton)
.Font(bold: true)
.CenterHorizontal()
.BindCommand(nameof(ViewModel.ClickMeButtonCommand)),
new Image { Source = "dotnet_bot.png", WidthRequest = 250, HeightRequest = 310 }
.Row(Row.Image).ColumnSpan(All<Column>())
.CenterHorizontal()
}
};
}
enum Row { HelloWorld, Welcome, Count, ClickMeButton, Image }
enum Column { Text, Number }
}
Contribute to .NET MAUI Toolkit
While the community toolkit libraries are built in collaboration with the .NET team at Microsoft, it is truly a community effort. The core team, Andrei Misiukevich, Pedro Jesus, Gerald Versluis, Javier Suárez, and (myself) Brandon Minnick, are here mostly to move things forward.
Your help and input is very much required. Whether that is through triaging issues, updating Docs, participating in discussions or adding actual code, we will need your help!
To learn how to contribute, check out "Contributing to .NET MAUI Community Toolkit": https://devblogs.microsoft.com/dotnet/contributing-to-net-maui-community-toolkit/