Upgrading to Refit v6.0 + Newtonsoft.Json
Refit recently released v6.0, replacing Newtonsoft.Json with System.Text.Json which may cause some breaking changes. Let's discuss how we can continue using Newtonsoft.Json with Refit!
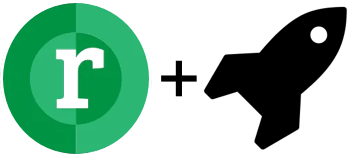
Quick Caveat
I do recommend using System.Text.Json because it has better performance than Newtonsoft.Json, and the .NET team continues to improve its performance in each new release of .NET:
That being said, migrating from Newtonsoft.Json to System.Text.Json is a breaking change and it may not be quick and easy depending on your code base.
Introducing Refit.Newtonsoft.Json
Along with Refit v6.0, the team released a new NuGet package, Refit.Newtonsoft.Json. This allows us to continue using Newtonsoft.Json as our default JSON serializer.
After adding the Refit.Newtonsoft.Json NuGet Package, here's how to use it:
Refit v5.0 (Old)
var gitHubApi = RestService.For<IGitHubApi>("https://api.github.com");
Refit v6.0 + Refit.Newtonsoft.Json (New)
var gitHubApi = RestService.For<IGitHubApi>("https://api.github.com", new RefitSettings(new NewtonsoftJsonContentSerializer(new JsonSerializerSettings { ContractResolver = new CamelCasePropertyNamesContractResolver() })););
That's it! We are now back to using Newtonsoft.Json as our default JSON serializer.
Refit.Newtonsoft.Json Extensions
Since the Refit.Newtonsoft.Json implementation is pretty verbose, I created the following helper methods to keep my code a bit simpler:
(Here's how I implemented this code in my open-source app GitTrends)
public static class RefitExtensions
{
public static T For<T>(string hostUrl) => RestService.For<T>(hostUrl, GetNewtonsoftJsonRefitSettings());
public static T For<T>(HttpClient client) => RestService.For<T>(client, GetNewtonsoftJsonRefitSettings());
public static RefitSettings GetNewtonsoftJsonRefitSettings() => new RefitSettings(new NewtonsoftJsonContentSerializer(new JsonSerializerSettings { ContractResolver = new CamelCasePropertyNamesContractResolver() }));
}
With the above RefitExtensions
, I can more easily initialize Refit in my client-side applications:
readonly static IGitHubApi _gitHubApi = RefitExtensions.For<IGitHubApi>("https://api.github.com");
And I can more easily initialize Refit in my on my backend applications with Refit.HttpClientFactory
:
public void ConfigureServices(IServiceCollection services)
{
services.AddRefitClient<IGitHubApi>(RefitExtensions.GetNewtonsoftJsonRefitSettings())
.ConfigureHttpClient(client => client.BaseAddress = new Uri("https://api.github.com"));
}
Conclusion
In the long term, I highly recommend migrating your apps to use System.Text.Json to benefit from its many performance improvements.
But in the short-term, Refit.Newtonsoft.Json is a great stop-gap to keep your current code running!
If you'd like to see how I implemented Refit.Newtonsoft.Json, here's how I use it in my open-source app, GitTrends: