Async Await Best Practices
Slides
Video Recording
Online Course
Join me in this 4 Hour DomeTrain course where we'll learn everything you need to know to master asynchronous programming using async await in C# and .NET:
From Zero to Hero: Asynchronous Programming in C#
Learn how to master asynchronous programming with async await in C#
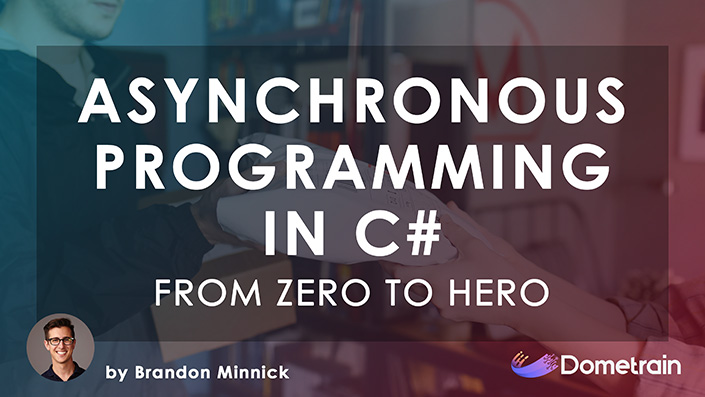
Source Code
NuGet Packages
AsyncAwaitBestPractices 9.0.0
Extensions for System.Threading.Tasks Includes extension methods to safely fire-and-forget a Task and/or a ValueTask Includes WeakEventManger which avoids memory leaks when events are not unsubscribed
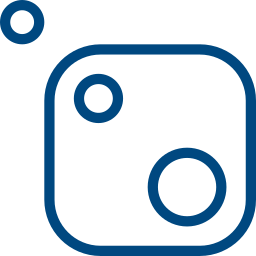
AsyncAwaitBestPractices.MVVM 9.0.0
Async Extensions for ICommand Includes AsyncCommand and IAsyncCommand which allows ICommand to safely be used asynchronously with Task. Includes AsyncValueCommand and IAsyncValueCommand which allows ICommand to safely be used asynchronously with ValueTask
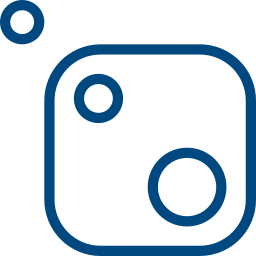
Learn .NET MAUI
From Zero to Hero: .NET MAUI
All the courses you need to learn and master .NET MAUI
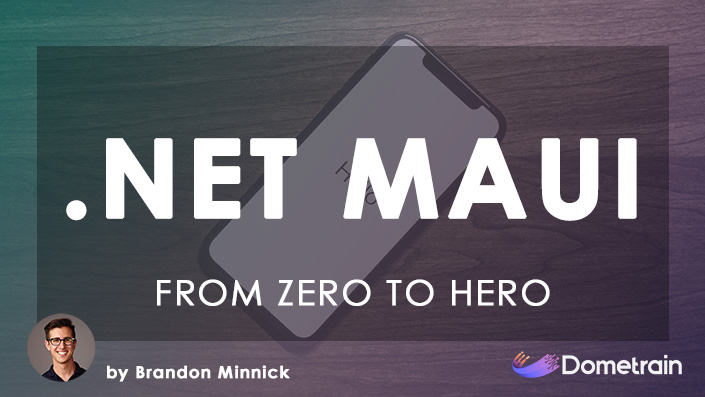
Additional Learning
Removing async void
When using async await we inevitably have to mark methods as async void. This leads to a lot of issues for programmers…
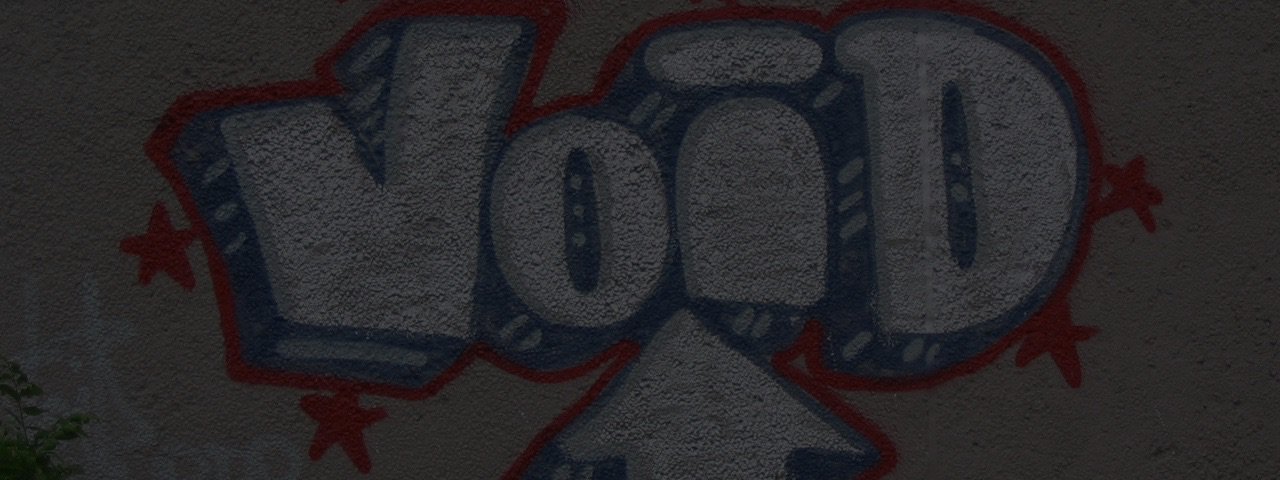
Asynchronous programming - C#
Explore an overview of the C# language support for asynchronous programming by using async, await, Task, and Task.

The managed thread pool - .NET
Learn about the .NET thread pool that provides background worker threads
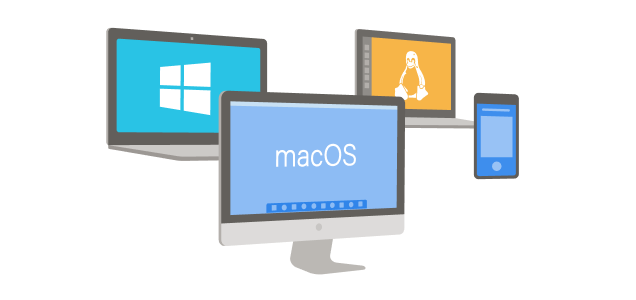
Understanding the Whys, Whats, and Whens of ValueTask - .NET Blog
The .NET Framework 4 saw the introduction of the System.Threading.Tasks namespace, and with it the Task class. This type and the derived Task<TResult> have long since become a staple of .NET programming, key aspects of the asynchronous programming model introduced with C# 5 and its async / await keywords. In this post, I’ll cover the newer ValueTask/ValueTask<TResult> types, which were introduced to help improve asynchronous performance in […]
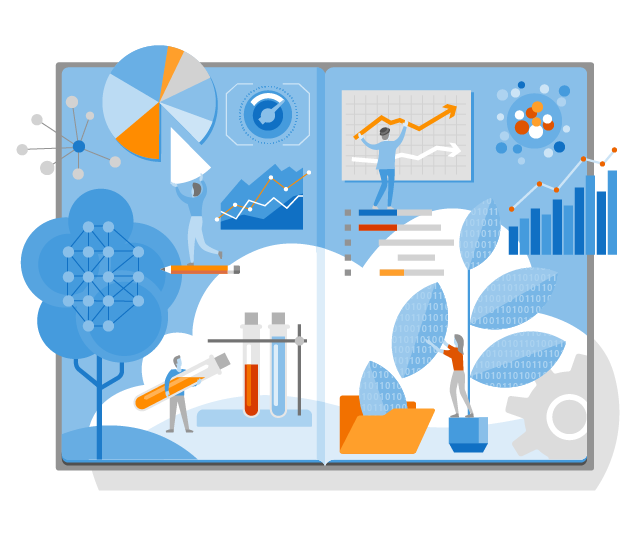
ExecutionContext vs SynchronizationContext - .NET Blog
I’ve been asked a few times recently various questions about ExecutionContext and SynchronizationContext, for example what the differences are between them, what it means to “flow” them, and how they relate to the new async/await keywords in C# and Visual Basic. I thought I’d try to tackle some of those questions here. WARNING: This post […]
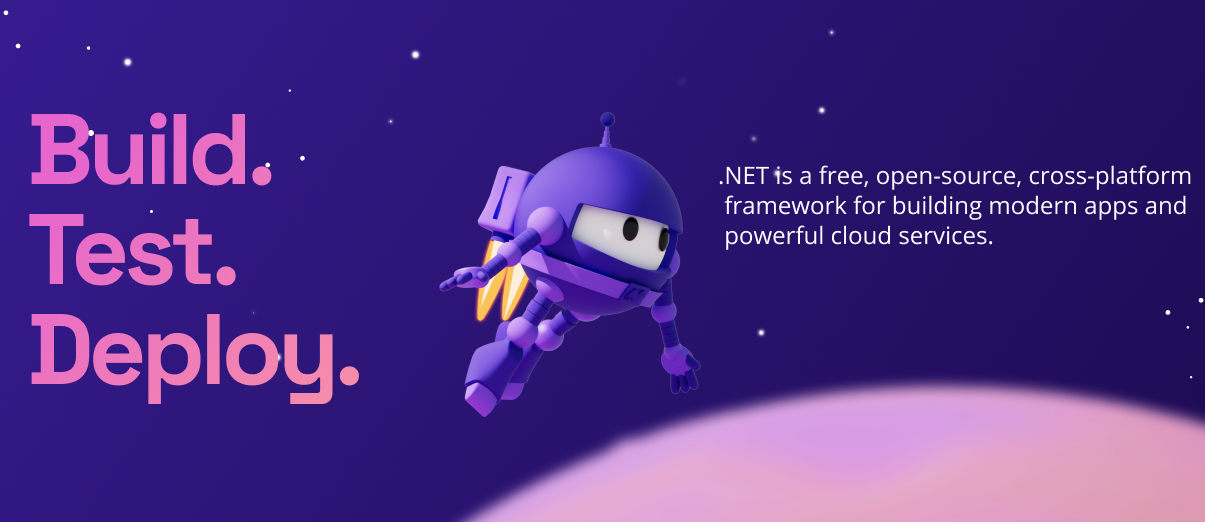
There Is No Thread
This is an essential truth of async in its purest form: There is no thread.
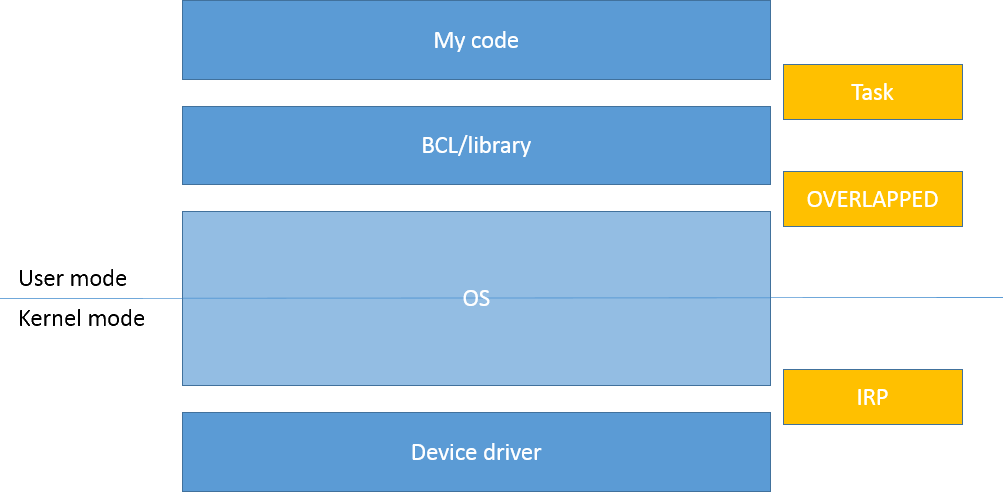
ConfigureAwait in .NET 8
Changes in ConfigureAwait that are new with .NET 8.0.
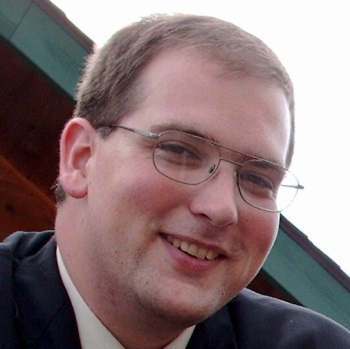
C# - Iterating with Async Enumerables in C# 8
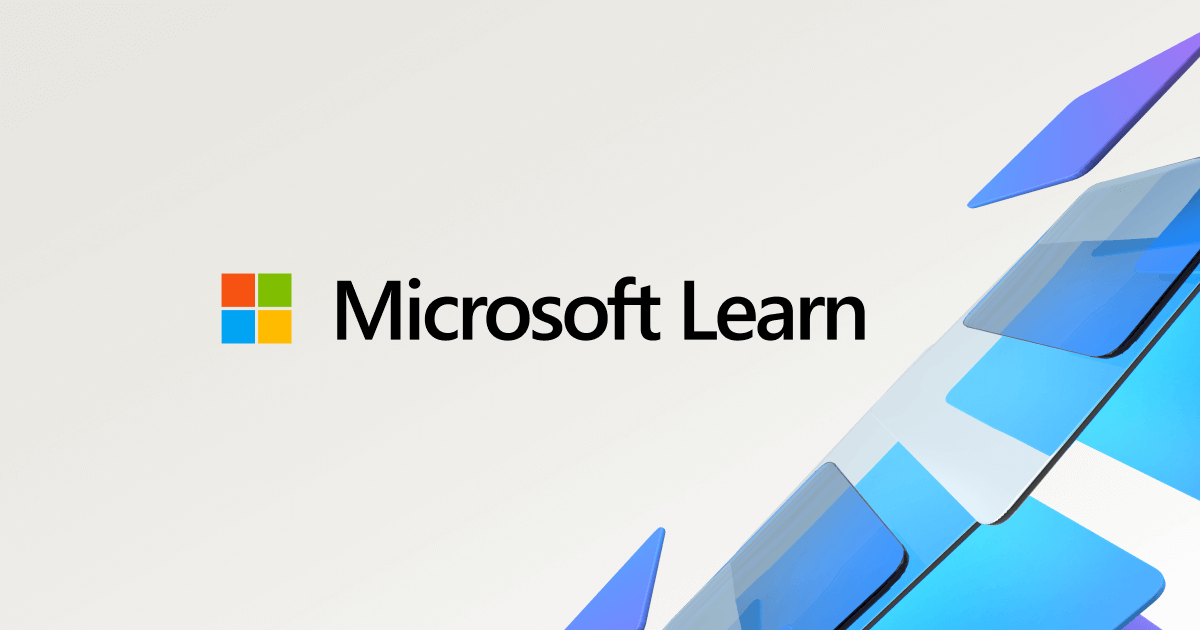
A deep-dive into the new Task.WaitAsync() API in .NET 6
In this post I look at how the new Task.WaitAsync() API is implemented in .NET 6, looking at the internal types used to implement it
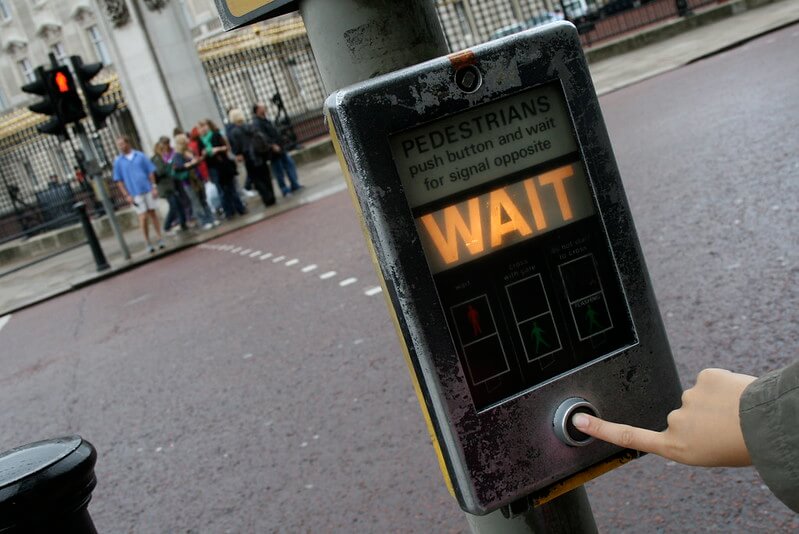
Using await using (IAsyncDisposable) with ConfigureAwait | tabs ↹ over ␣ ␣ ␣ spaces by Jiří {x2} Činčura

Execution Context Management with AsyncLocal and ThreadLocal in .NET Core
Introduction In modern distributed .NET applications, managing context across execution boundaries is a critical architectural concern. While both AsyncLocal<T> and ThreadLocal<T> provide mechanisms for maintaining contextual data, their implementations and use cases differ significantly in ways that impact system architecture, performance, and maintainability. This comprehensive guide explores both
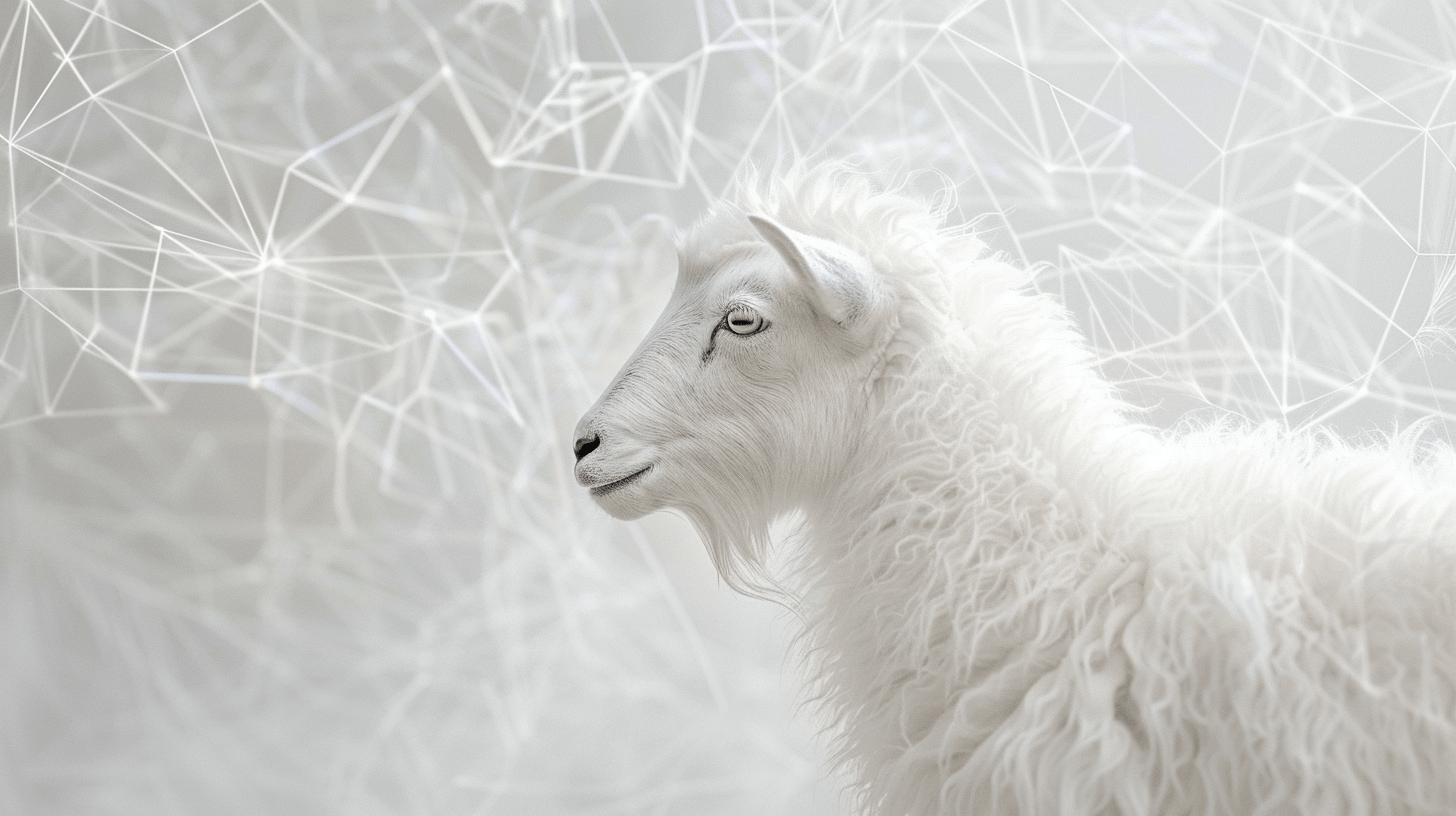